Top 50 JUnit Interview Question and Answers
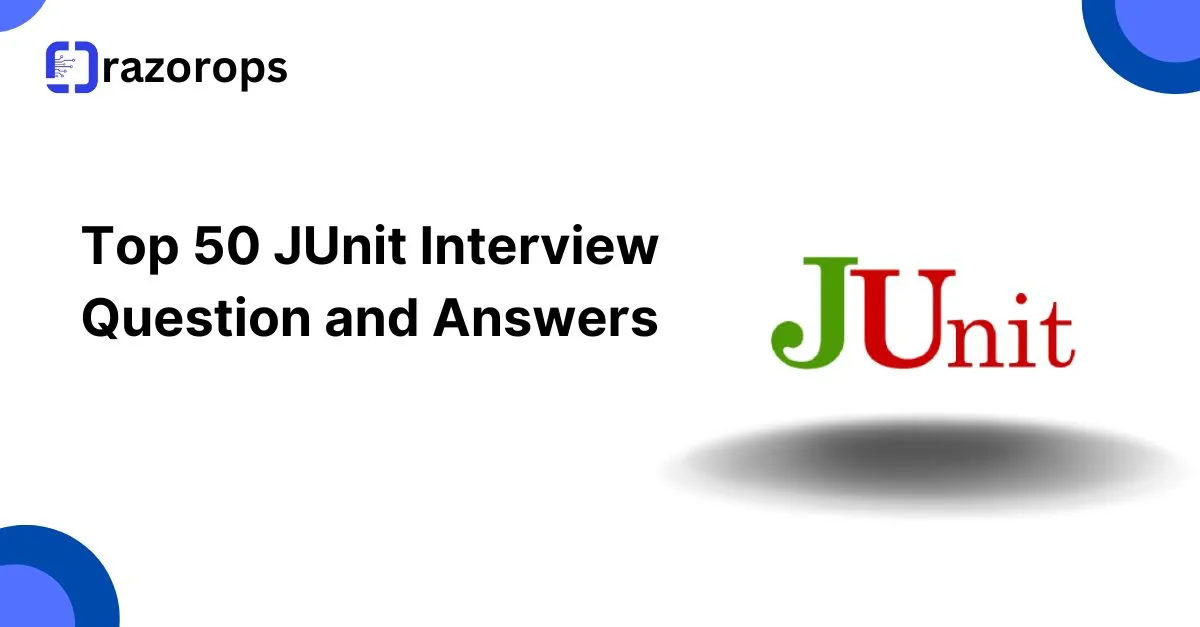
-
What is JUnit?
- JUnit is a popular Java testing framework used for unit testing. It provides annotations to identify test methods, asserts for verifying expected results, and integration with tools like Maven or Gradle.
-
Why is JUnit important?
- JUnit automates testing, ensures code reliability, promotes TDD (Test-Driven Development), and integrates easily with CI/CD pipelines.
-
Explain the lifecycle of a JUnit test.
- Lifecycle includes:
-
@BeforeAll
(setup for all tests, runs once). -
@BeforeEach
(setup before each test). - Test methods (
@Test
). -
@AfterEach
(cleanup after each test). -
@AfterAll
(cleanup for all tests, runs once).
-
- Lifecycle includes:
-
What are some key annotations in JUnit?
- Common annotations:
-
@Test
: Marks a test method. -
@BeforeAll
/@BeforeEach
: Setup methods. -
@AfterAll
/@AfterEach
: Teardown methods. -
@Disabled
: Disables a test.
-
- Common annotations:
-
How does JUnit assert equality?
- Using methods like:
-
assertEquals(expected, actual)
. -
assertNotEquals(expected, actual)
.
-
- Using methods like:
-
What is the difference between
@BeforeEach
and@BeforeAll
?-
@BeforeEach
runs before every test, while@BeforeAll
runs once before all tests.
-
-
What is
assertThrows()
in JUnit 5?- Validates that a specific exception is thrown during test execution.
assertThrows(IllegalArgumentException.class, () -> { throw new IllegalArgumentException("Invalid argument"); });
-
How do you handle timeouts in JUnit tests?
- Use the
@Test(timeout = ms)
annotation in JUnit 4 or@Test
withassertTimeout
in JUnit 5.
- Use the
-
What is the difference between
assertAll
and individual asserts?-
assertAll
allows multiple assertions to execute even if one fails, collecting all errors.
-
-
How do you disable a test in JUnit?
- Annotate the method with
@Disabled
.
- Annotate the method with
-
How can you run tests in a specific order?
- Use
@TestMethodOrder(OrderAnnotation.class)
and@Order(n)
annotations in JUnit 5.
- Use
-
Explain parameterized tests in JUnit.
- Allows running the same test with different data inputs using
@ParameterizedTest
.
- Allows running the same test with different data inputs using
-
What is the role of
@ExtendWith
?- Used to integrate extensions like Mockito, Spring, or custom behaviors into JUnit tests.
-
How does JUnit support dependency injection?
- Using
@ParameterizedTest
and@ValueSource
or integration with external frameworks like Spring.
- Using
-
What is JUnit’s role in TDD?
- TDD relies heavily on frameworks like JUnit to write failing tests before implementing functionality.
-
How do you integrate JUnit with Maven?
- Add the JUnit dependency in the `pom.xml` file:
xml
`<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.x.x</version>
<scope>test</scope>
</dependency>`
- What is the difference between JUnit 4 and JUnit 5?
- JUnit 5 uses a modular architecture with better extension support, Java 8+ features, and new annotations like `@BeforeAll`/`@AfterAll`. 18. **How do you test private methods with JUnit?**
- Use reflection or redesign code to test through public interfaces. 19. **How do you generate JUnit test reports?**
- Use plugins like Surefire or Gradle to generate HTML/XML reports. 20. **What is the difference between `@Mock` and `@Spy` in JUnit with Mockito?**
- `@Mock`: Creates a mock object.
- `@Spy`: Partially mocks an object, retaining real behavior.
- What is the difference between
assertArrayEquals
andassertIterableEquals
?
-
assertArrayEquals
compares arrays element by element to ensure they are equal. -
assertIterableEquals
compares two iterable objects (e.g., lists) for equality.
Example:
assertArrayEquals(new int[]{1, 2}, new int[]{1, 2}); // Arrays
assertIterableEquals(List.of(1, 2), List.of(1, 2)); // Lists
- What are assumptions in JUnit?
- Assumptions allow tests to execute conditionally. If an assumption fails, the test is skipped.
Example:
@Test
void testOnSpecificOS() {
assumeTrue(System.getProperty("os.name").contains("Windows"));
// Test code that runs only on Windows
}
- How do you disable tests conditionally in JUnit 5?
- Use annotations like
@EnabledOnOs
or@DisabledOnOs
for OS-specific conditions, or@EnabledIf
for custom conditions.
Example:
@EnabledOnOs(OS.WINDOWS)
@Test
void windowsOnlyTest() {
assertTrue(true);
}
- What is the
assertThat
method in JUnit?
-
assertThat
provides advanced assertions using Hamcrest matchers, enabling more readable test conditions.
Example:
assertThat("JUnit", containsString("Unit"));
- How do you write repeated tests in JUnit 5?
- Use the
@RepeatedTest
annotation to run a test multiple times.
Example:
@RepeatedTest(5)
void repeatedTest() {
System.out.println("This test runs 5 times");
}
- What is the purpose of the
@ParameterizedTest
annotation?
- It allows testing with multiple sets of data inputs without duplicating code.
Example:
@ParameterizedTest
@ValueSource(ints = {1, 2, 3})
void testWithMultipleInputs(int number) {
assertTrue(number > 0);
}
- How do you use
@MethodSource
in parameterized tests?
-
@MethodSource
provides data for parameterized tests from a static method.
Example:
`static Stream
@ParameterizedTest @MethodSource(“provideData”) void testWithMethodSource(int a, int b, int result) { assertEquals(result, a + b); }`
- What is the purpose of the
@TestFactory
annotation?
- It is used to create dynamic tests at runtime instead of static, predefined test methods.
Example:
@TestFactory
Collection<DynamicTest> dynamicTests() {
return List.of(
DynamicTest.dynamicTest("Test 1", () -> assertTrue(true)),
DynamicTest.dynamicTest("Test 2", () -> assertEquals(4, 2 + 2))
);
}
- How can you group tests in JUnit 5?
- Use
@Nested
for hierarchical grouping and@Tag
for categorization of tests.
Example with @Nested
:
@Nested
class MathTests {
@Test
void additionTest() {
assertEquals(5, 2 + 3);
}
}
Example with @Tag
:
@Tag("slow")
@Test
void slowTest() {
assertTrue(true);
}
- What are test suites in JUnit?
- Test suites group multiple test classes and execute them together.
- JUnit 5 doesn’t provide built-in test suites but relies on tools like Maven Surefire or Gradle.
- How do you use
@Nested
tests in JUnit?
-
@Nested
organizes related test cases within a class. It improves test readability and structure.
@Nested
class InnerClassTest {
@Test
void testMethod() {
assertTrue(true);
}
}
- How do you mock objects in JUnit?
- Use frameworks like Mockito with
@Mock
to create mock objects and verify interactions.
@Mock
private MyService service;
- What is the difference between
doReturn
andthenReturn
in Mockito?
-
doReturn
is used for stubbing methods with void return types, whilethenReturn
is used for non-void return types.
- How do you mock static methods in JUnit?
- Use frameworks like PowerMock or Mockito’s
mockStatic
introduced in Mockito 3.4.
- How do you verify method calls in JUnit?
-
Use Mockito’s
verify
method:verify(mockService).methodName();
- What is
@Captor
in Mockito?
- It is used to capture arguments passed to a mocked method.
- How do you write tests for interfaces?
- Test the implementations of the interface or mock the interface and verify interactions.
- What are assertions in JUnit, and why are they important?
- Assertions validate the expected output of the test. Examples include
assertTrue
,assertFalse
, andassertEquals
.
- How do you write parameterized tests for multiple input combinations?
- Use
@CsvSource
or@CsvFileSource
with@ParameterizedTest
.
@ParameterizedTest
@CsvSource({"1, 2, 3", "4, 5, 9"})
void testAddition(int a, int b, int expected) {
assertEquals(expected, a + b);
}
- What is
@Timeout
in JUnit 5?
- It ensures a test does not exceed the specified execution time. If it does, the test fails.
@Test
@Timeout(1)
void testWithTimeout() throws InterruptedException {
Thread.sleep(500);
}
- What is the role of
@Condition
in JUnit?
- Allows conditional test execution based on system properties, OS, or environment variables using
@EnabledIf
or@DisabledIf
.
- How do you integrate JUnit with Gradle?
-
Add the JUnit dependency in
build.gradle
:testImplementation 'org.junit.jupiter:junit-jupiter:5.x.x'
- How can JUnit tests be run in CI/CD pipelines?
- Integrate JUnit with Jenkins, GitHub Actions, or GitLab CI/CD to execute tests as part of the build process.
- What plugins are used to generate reports for JUnit?
- Use Maven Surefire Plugin or Gradle Test Report for generating HTML/XML test reports.
- What is the purpose of
assertJ
in JUnit?
- AssertJ provides a fluent API for assertions, making tests more readable.
- Can JUnit be used for performance testing?
- JUnit itself is not ideal for performance testing but can integrate with tools like JMH (Java Microbenchmark Harness).
- How do you test exception scenarios in JUnit?
- Use
assertThrows
for verifying expected exceptions.
assertThrows(NullPointerException.class, () -> {
throw new NullPointerException();
});
- What is the purpose of
assertDoesNotThrow
?
- Validates that a block of code does not throw any exception.
- How do you mock void methods in JUnit?
- Use Mockito’s
doNothing
ordoThrow
methods.
doNothing().when(mockService).methodName();
- What is the role of
@Tag
in JUnit?
- Tags categorize test cases, enabling selective execution.
@Tag("IntegrationTest")
@Test
void testIntegration() {
assertTrue(true);
}
- How do you test collections in JUnit?
- Use
assertIterableEquals
orassertArrayEquals
.