Top 50 GitHub Actions Interview Question and Answers
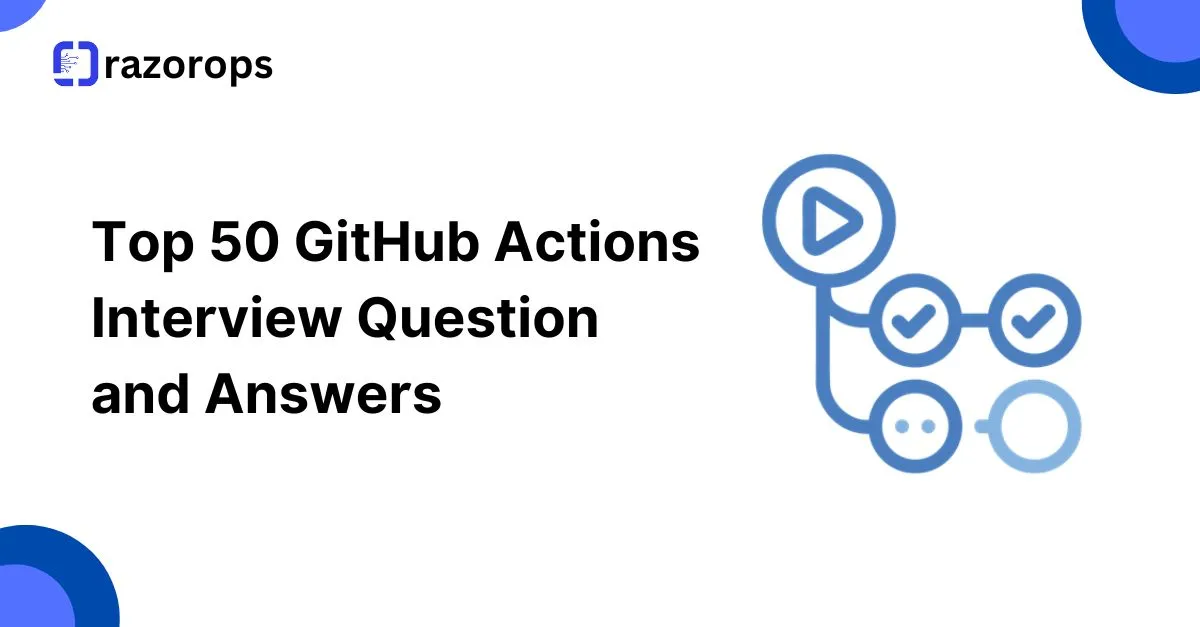
-
What is GitHub Actions?
- GitHub Actions is a CI/CD platform that enables automation directly within GitHub repositories for building, testing, and deploying code.
-
How do workflows work in GitHub Actions?
- Workflows are automated processes defined in YAML files under
.github/workflows
and are triggered by specific events, such aspush
orpull_request
.
- Workflows are automated processes defined in YAML files under
-
What are jobs in GitHub Actions?
- Jobs are individual tasks that run within workflows. Each job can include multiple steps and can run in parallel or sequentially.
-
Explain steps in GitHub Actions.
- Steps are the individual tasks executed in a job. They can be custom shell commands or actions.
-
What are runners in GitHub Actions?
- Runners are servers that execute workflows. GitHub provides hosted runners, or you can use self-hosted runners for custom environments.
-
What’s the difference between GitHub-hosted and self-hosted runners?
- GitHub-hosted runners are managed by GitHub and provide common tools. Self-hosted runners are managed by users for custom configurations.
-
How do you define a workflow trigger in GitHub Actions?
- Workflow triggers are defined using the
on
keyword, specifying events likepush
,pull_request
, orschedule
.
- Workflow triggers are defined using the
-
What are reusable workflows in GitHub Actions?
- Reusable workflows allow common tasks to be centralized in one workflow file and invoked in multiple repositories.
-
What are artifacts in GitHub Actions?
- Artifacts are files generated by workflows (e.g., build files) that can be saved and shared across jobs or with users.
-
How do you manage secrets in GitHub Actions?
- Secrets are stored in GitHub repository settings and accessed in workflows using
$
syntax.
- Secrets are stored in GitHub repository settings and accessed in workflows using
-
How can you run a job only on specific branches?
- Specify branches under the
on
condition, e.g.,on: push: branches: [main, dev]
.
- Specify branches under the
-
What is a matrix strategy in GitHub Actions?
- Matrix strategy runs a job multiple times with different configurations (e.g., OS, Node.js versions).
-
Explain how caching works in GitHub Actions.
- GitHub Actions supports caching dependencies to speed up workflow execution using the
actions/cache
action.
- GitHub Actions supports caching dependencies to speed up workflow execution using the
-
What is
needs
in GitHub Actions?-
needs
is used to define job dependencies, ensuring jobs run sequentially when necessary.
-
-
How do you add conditional logic to GitHub Actions workflows?
- Use
if
statements to define conditions, such asif: success()
orif: always()
.
- Use
-
What is the purpose of
continue-on-error
?-
continue-on-error: true
allows a job to proceed even if a step fails.
-
-
What is a composite action?
- A composite action bundles multiple steps in one action, making it reusable.
-
How do you pass environment variables across jobs?
- Use
env
for global or job-level variables or pass them as outputs between jobs.
- Use
-
How do you debug workflows in GitHub Actions?
- Use
::debug::
commands, review logs, or set upACTIONS_RUNNER_DEBUG
in secrets for verbose logging.
- Use
-
How do you run scheduled workflows?
- Use
on: schedule
with a cron syntax (e.g.,cron: '0 0 * * 1'
for weekly triggers).
- Use
-
How do you reuse workflows across repositories?
- Use reusable workflows by referencing them in other repositories with the
uses
syntax.
- Use reusable workflows by referencing them in other repositories with the
-
How do you handle secrets in workflows safely?
- GitHub Actions masks secrets automatically and restricts them from appearing in logs.
-
What is the purpose of
job.container
?-
job.container
allows running jobs inside a Docker container for isolated environments.
-
-
What is
jobs.<job_id>.outputs
?- Outputs are values that can be set in a job and accessed by dependent jobs.
-
Explain the purpose of the
env
keyword.-
env
defines environment variables for steps, jobs, or workflows.
-
-
How can you use a Docker image in GitHub Actions?
- Define
container: image: <docker-image>
in the job or use Docker commands in steps.
- Define
-
How do you specify a particular runner version?
- Choose an OS version (e.g.,
runs-on: ubuntu-latest
orruns-on: windows-2019
).
- Choose an OS version (e.g.,
-
What’s the purpose of
jobs.<job_id>.timeout-minutes
?- This sets a timeout for the job, after which it’s automatically canceled.
-
What is a service container?
- Service containers are additional containers run alongside jobs, useful for databases or message queues.
-
What’s the difference between
actions/checkout@v2
andactions/checkout@v3
?- Each major version may introduce performance improvements and bug fixes; v3 includes enhancements over v2.
-
What are custom actions?
- Custom actions are user-defined tasks created in JavaScript or Docker that can be reused across workflows.
-
How do you use GitHub Actions with multiple repositories?
- Use
checkout
with repository names or trigger workflows across repos usingrepository_dispatch
.
- Use
-
What is concurrency in GitHub Actions?
- Concurrency controls simultaneous runs of workflows, allowing them to cancel previous runs if needed.
-
How can you prevent secrets from leaking?
- Mask secrets in logs, avoid
set-output
with secrets, and usesecrets
safely in workflows.
- Mask secrets in logs, avoid
-
How do you handle GitHub Actions permissions?
- Set granular permissions for actions in the repository settings or
permissions
keyword in workflows.
- Set granular permissions for actions in the repository settings or
-
What is the purpose of
GITHUB_TOKEN
?-
GITHUB_TOKEN
is an auto-generated token providing access to the repo for secure API interactions.
-
-
What is
jobs.<job_id>.strategy.fail-fast
?- It stops all jobs in a matrix if any job fails, reducing resource use.
-
How do you manage workflow concurrency?
- Use
concurrency
to limit simultaneous runs or cancel redundant runs withcancel-in-progress
.
- Use
-
What are required status checks in GitHub Actions?
- These are checks that must pass before merging, typically set in branch protection rules.
-
Explain the purpose of
jobs.<job_id>.defaults
.-
defaults
provides defaultrun
settings for steps in a job.
-
-
How do you handle errors in GitHub Actions?
- Use
if: failure()
for error handling, or addcontinue-on-error
for steps that shouldn’t block progress.
- Use
-
What are the best practices for securing workflows?
- Avoid exposing secrets, validate inputs, and use reusable actions from trusted sources.
-
How can you optimize GitHub Actions for performance?
- Use caching, parallel jobs, matrix builds, and reusable workflows.
-
How do you troubleshoot failed workflows?
- Check logs, enable debug logging, and use the
::debug::
command.
- Check logs, enable debug logging, and use the
-
What’s the purpose of
jobs.<job_id>.env
?-
env
at the job level sets environment variables available to all steps in that job.
-
-
How do you create a GitHub Action using JavaScript?
- Create an action.yml file, define inputs, and use JavaScript to build the action logic.
-
What’s the role of
jobs.<job_id>.if
?-
if
allows conditional job execution based on specific criteria.
-
-
What is
on.workflow_call
?-
on.workflow_call
triggers a reusable workflow, allowing it to be called from other workflows.
-
-
How can you share artifacts across jobs?
- Use
upload-artifact
to save files in one job anddownload-artifact
to access them in another.
- Use
-
What are the benefits of GitHub Actions over other CI/CD tools?
- GitHub Actions provides native GitHub integration, reusable workflows, strong community support, and flexible automation.